-
Django Signals Dashboard
Forum: Algorithmic Trading With Python
Last Post: Hassam
12-14-2023, 02:00 PM
» Replies: 2
» Views: 0 -
Trading Journal
Forum: Million Dollar Trading Challenge
Last Post: Hassam
11-07-2023, 01:29 PM
» Replies: 40
» Views: 51 -
MQL4 Script to read the M...
Forum: MQL4
Last Post: Hassam
04-22-2023, 01:43 PM
» Replies: 0
» Views: 0 -
MACD_Color Indicator MQL5...
Forum: MQL5
Last Post: Hassam
10-03-2022, 09:46 AM
» Replies: 1
» Views: 0 -
Crypto & On-Chain Analysi...
Forum: Quantitative Trading Strategies
Last Post: patryknextdoor
04-28-2022, 06:59 PM
» Replies: 13
» Views: 0 -
Analysis of Patterns in T...
Forum: Quantitative Trading Strategies
Last Post: Hassam
04-08-2022, 01:00 PM
» Replies: 7
» Views: 0 -
Single Currency Measureme...
Forum: Quantitative Trading Strategies
Last Post: patryknextdoor
04-07-2022, 08:04 AM
» Replies: 13
» Views: 0 -
Conditional Scoring and R...
Forum: Quantitative Trading Strategies
Last Post: Hassam
04-04-2022, 06:06 AM
» Replies: 1
» Views: 0 -
FOREX TRADING SUCCESS
Forum: Quantitative Trading Strategies
Last Post: patryknextdoor
04-02-2022, 02:20 AM
» Replies: 8
» Views: 0 -
Order Flow/Limit Order Bo...
Forum: Quantitative Trading Strategies
Last Post: patryknextdoor
03-29-2022, 07:34 AM
» Replies: 0
» Views: 0
- Forum posts:226
- Forum threads:59
- Members:13,493
- Latest member:g6syqjd295
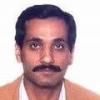
In this project, I will built a Django Dashboard that is connected with MT4 and MT5. I will post signals on Django Dashboard. MT4 SignalsEA and MT5 SignalsEA will read these signals and open and closed trades on MT4 and MT5 and in return inform Django that the signals have been successfully copied or their was an error. This Django Dashboard front end will be in React. So I will be easily able to open it on my mobile phone. The idea is the use the mobile phone to open and close signals and monitor multiple MT4 and MT5 accounts that can be upto 50-100. First I created SignalDashboard project folder and then I create a backend folder in it and from this folder I launched virtual environment:
python -m venv venv
.\venv\Scripts\activate
First I install virtual environment and then I activated it. Now create a text file in SignalDashboard/bankend folder and add:
Django==4.0
Now install Django:
pip install -r requirements.txt
Now create the backend project:
django-admin startproject backend .
For now we will be using sqlite3. Run migrations:
python manage.py migrate
Install Django Rest Framework:
pip install djangorestframework
Now create the signals app:
python manage.py startapp signals
These are useful shell commands
# python manage.py dbshell
#python manage.py flush
#PRAGMA table_info(SignalEA_signalea);
#python manage.py shell
#python manage.py makemigrations
#python manage.py migrate
#python manage.py runserver
#python manage.py startapp Signal
#python manage.py validate
Create an app signals within the backend folder.
python manage.py startapp signals
Now define the model in model.py of the signals and then enter that in admin.py and make entry in the backend settings.py telling about the restframework and signals app.
admin.site.register(Signal)
You also need to tell urls.py in backend to check urls.py in signals app.
path('signals/', include('signals.urls')
Then you have to make serializers.py file in signals app and make a serializers class. After that you have views .py.
Now I made the models. One is Signal. I have generated this JSON object when running the server on localhost:
http://127.0.0.1:8000/signals/signals?format=json
{"signals":[{"id":1,"signal":"buylimit\r\nGBPUSD\r\n240\r\n1\r\n0\r\n3\r\n2\r\nLiteFinance"},{"id":2,"signal":"selllimit\r\nEURUSD\r\n240\r\n0\r\n0\r\n3\r\n1"},{"id":3,"signal":"selllimit\r\nEURNZD\r\n240\r\n2\r\n0\r\n3\r\n1"}]}
These are the request headers:
Accept
text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8
Accept-Encoding
gzip, deflate, br
Accept-Language
en-US,en;q=0.5
Connection
keep-alive
Host
127.0.0.1:8000
Referer
http://127.0.0.1:8000/signals/signals?format=api
Sec-Fetch-Dest
document
Sec-Fetch-Mode
navigate
Sec-Fetch-Site
same-origin
Sec-Fetch-User
?1
Upgrade-Insecure-Requests
1
User-Agent
Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/119.0
These are the response headers:
Allow
GET, HEAD, OPTIONS
Content-Length
228
Content-Type
application/json
Cross-Origin-Opener-Policy
same-origin
Date
Tue, 07 Nov 2023 13:51:57 GMT
Referrer-Policy
same-origin
Server
WSGIServer/0.2 CPython/3.8.8
Vary
Accept, Cookie
X-Content-Type-Options
nosniff
X-Frame-Options
DENY
When I try to use Webrequest on MT4. It gives an invalid array error. I need to use Wireshark to check what is happening.
Frame 196: 55 bytes on wire (440 bits), 55 bytes captured (440 bits) on interface \Device\NPF_Loopback, id 0
Null/Loopback
Internet Protocol Version 4, Src: 127.0.0.1, Dst: 127.0.0.1
Transmission Control Protocol, Src Port: 35600, Dst Port: 53338, Seq: 173, Ack: 196, Len: 11
[3 Reassembled TCP Segments (158 bytes): #192(17), #194(130), #196(11)]
Hypertext Transfer Protocol
HTTP/1.0 200 OK\r\n
Content-Type: application/json\r\n
Content-Length: 11\r\n
Server: Werkzeug/0.16.0 Python/3.11.3\r\n
Date: Wed, 08 Nov 2023 04:19:11 GMT\r\n
\r\n
[HTTP response 2/2]
[Time since request: 0.001118000 seconds]
[Prev response in frame: 188]
[Request in frame: 190]
[Request URI: http://127.0.0.1:35600/api/v2/api_lock]
File Data: 11 bytes
JavaScript Object Notation: application/json
Object
Before you open a trade, you need to have the amount greater than the margin required for trading that currency pair. Below is the MQL4 script that you can use to easily check the margin required for that currency pair and the spread for that currency pair:
//+------------------------------------------------------------------+
//| Margin.mq4 |
//| Ahmad Hassam |
//| https://www.doubledoji.com |
//+------------------------------------------------------------------+
#property copyright "Ahmad Hassam"
#property link "https://www.doubledoji.com"
#property version "1.00"
#property strict
//+------------------------------------------------------------------+
//| Script program start function |
//+------------------------------------------------------------------+
void OnStart()
{
//---
Print("Symbol=",Symbol());
Print("Spread value in points=",MarketInfo(Symbol(),MODE_SPREAD));
Print("Free margin required to open 1 lot for buying=",MarketInfo(Symbol(),MODE_MARGINREQUIRED));
}
//+------------------------------------------------------------------+
I use MACD a lot in my trading. I use MACD as a momentum indicator. I need to now when the momentum is becoming strong and when the momentum is becoming weak. The default MACD indicator provided on MT5 has no color. So I have coded a new indicator that uses the default MT5 MACD indicator and colors the bar. When the momentum is strong in up direction it will color the bar as green and when the momentum is strong in the down direction it will color the bar in red. When the momentum increase and the MACD is below zero the bar will be light green and when the momentum increases and MACD is above zero, the bar will be dark green. In the same manner, when the momentum increases in down direction the MACD is above zero, bar color will be light red and when momentum increases in down direction and MACD is below zero, bar color will be dark red. Below is the MQL5 code for this MACD_Color Indicator:
//+------------------------------------------------------------------+
//| MACD_Color.mq5 |
//| Copyright 2019, Ahmad Hassam |
//| https://www.doubledoji.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2019, Ahmad Hassam"
#property link "https://www.doubledoji.com"
#property version "1.00"
#property description "Moving Average Convergence/Divergence"
#property indicator_separate_window
#property indicator_buffers 4
#property indicator_plots 2
//--- plot MACD HISTOGRAM
#property indicator_label1 "MACD"
#property indicator_type1 DRAW_COLOR_HISTOGRAM
#property indicator_color1 clrLightGreen, clrDarkGreen, clrPink, clrRed
#property indicator_style1 STYLE_SOLID
#property indicator_width1 3
//--- plot MACD Signal
#property indicator_label2 "Signal"
#property indicator_type2 DRAW_LINE
#property indicator_color2 clrRed
#property indicator_style2 STYLE_SOLID
#property indicator_width2 2
//--- input parameters
input int fastEMA=12;
input int slowEMA=26;
input int signalPeriod=9;
input ENUM_APPLIED_PRICE MAPrice = PRICE_CLOSE;
//--- indicator buffers
double macd_main[]; //MACD Histogram
double macd_color[]; //MACD Histogram bar colors
double macd_signal[]; //MACD Signal
double macd_signal_color[];
//--declare MACD Handle
int macdHandle;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- indicator subwindow label
IndicatorSetString(INDICATOR_SHORTNAME,
"MACD("+string(fastEMA)+", "+string(slowEMA)+","+string(signalPeriod)+")");
//--- indicator buffers mapping
//--- Bind the color buffer immediately below the data buffer
SetIndexBuffer(0,macd_main,INDICATOR_DATA);
ArraySetAsSeries(macd_main,true);
SetIndexBuffer(1,macd_color,INDICATOR_COLOR_INDEX);
ArraySetAsSeries(macd_color,true);
SetIndexBuffer(2,macd_signal,INDICATOR_DATA);
ArraySetAsSeries(macd_signal,true);
SetIndexBuffer(3,macd_signal_color,INDICATOR_COLOR_INDEX);
ArraySetAsSeries(macd_signal_color,true);
//--Initialize the MACD
macdHandle = iMACD(_Symbol,_Period,fastEMA,
slowEMA,signalPeriod, MAPrice);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
int end;
if(prev_calculated < rates_total)
end=rates_total - prev_calculated - 2;
else
end = 1;
//--- Data preparation
CopyBuffer(macdHandle,0,0,end+2,macd_main);
CopyBuffer(macdHandle,1,0,end+2,macd_signal);
//---
for(int i = 0; i < end; i++)
{
//--color code the MACD bars
if( macd_main[i] < 0 && macd_main[i] > macd_main[i+1])macd_color[i]=0;
else if( macd_main[i] > 0 && macd_main[i] > macd_main[i+1])macd_color[i]=1;
else if( macd_main[i] > 0 && macd_main[i] < macd_main[i+1])macd_color[i]=2;
else if( macd_main[i] < 0 && macd_main[i] < macd_main[i+1])macd_color[i]=3;
}
//--- return value of prev_calculated for next call
return(rates_total);
}
//+------------------------------------------------------------------+
//| Indicator deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
if(macdHandle!=INVALID_HANDLE)
IndicatorRelease(macdHandle);
//--- clear the chart after deleting the indicator
Comment("");
}
//+------------------------------------------------------------------+
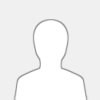
Do you think if its possible to create crypto currency strength meter?
Do you have experience with on chain analysis in crypto trading?
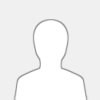
Hallo Sir!
It can be hard , time consuming sometimes to manually look at each currency pair and look for trading opportunities ,especially in short term trading.
Sometimes it can make someone to loose opportunities because 'you were too late' or sometimes one can be tired or have a bad day and won't analyze properly which leads to losing trades. Sometimes someone wants to focus only on Major pairs meanwhile there is much greater opportunity on Exotic pairs and the person doesn't know.
I think there could be a solution to that problem called Scoring and Ranking System based on some predefined evaluated conditions.
I believe such basic system would make it easier to avoid loosing opportunities , would save time and could help to filter out more potential trades which could be more accurate.
Could you teach me how to build such Program in Python that as a result would screen and filter out pairs that are most likely to increase/decrease in price OR to be more precise , screen the potential for trading opportunities based on predefined concepts ,before taking a position in the market?
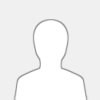
I wanted to start this thread so we can gather ideas together and work on Order Flow and Limit Order Book Analysis in realm time with Python or any other appropriate tools.
Let me know your view on that case and if you have any ideas yourself.
I will be commenting my ideas as well as long as we go.
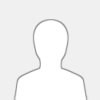
This question is on my mind very much...
Is it a good idea to create currency strength meter and then apply and fit a linear model (as indicator) around this single currency strength and use it in actual trading to predict price/path from that individual currency strength?
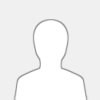
As you agreed with me that pattern does not mean the well known chart patterns. Patterns means time series patterns that have some statistical property that can be measured.
Can you teach me how to extract and interpret useful information/patterns from time series with some statistical measurable property for trading?
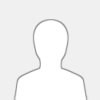
Imagine i start with 1000 dollar account and i risk 1 % for a trade which is 10 dollar and i want to make 200 dollar ratio reward.
Most of time i would get stopped out easily by the market with such tight stop loss or if my lot size is bigger but still its not excessing the 1 % risk ratio.
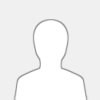
Dear Ahmad,
As mentioned on facebook you did suggest to teach ,so let me keep it simple in those questions below:
1. Can you to teach me how to successfully trade & make money trading forex ?(scalping, daytrading and swing trading)
2. How to create ''home made'' trading system and which tools to use to improve my decision making in trading and gaining some edge?
3.Can you teach me to analyse order flow and limit order book behaviour with python in real time?
4. How to understand market behaviour/dynamics and data generation process in the fx market?
I am very motivated and ambitious to learn no matter what you will direct me to learn i will go and i will learn it.
Thank You in advance!
Best regards,
Patryk